Searching Algorithms
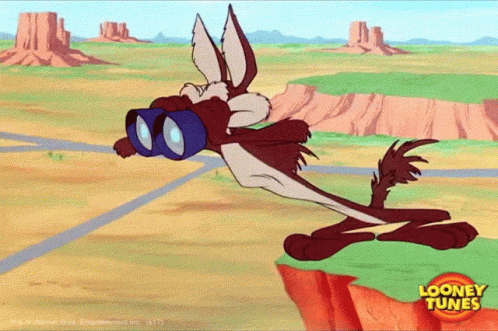
Definition
- Searching algorithms are designed to find an element in a list or array.
- The most common searching algorithms are:
- Linear Search
- Binary Search
Linear Search
- Linear search is the simplest searching algorithm.
- It sequentially checks each element of the list until a match is found or the whole list has been searched.
Code
Explanation
- The function
linearSearch
takes an arrayarr
, the size of the arrayn
, and the element to be searchedx
as input. - It iterates through each element of the array and compares it with the element to be searched.
- If a match is found, it returns the index of the element. Otherwise, it returns
-1
.
Analysis
- Time Complexity:
O(n)
wheren
is the number of elements in the array. - Space Complexity:
O(1)
as no extra space is used.
Binary Search
- Binary search is a more efficient searching algorithm for sorted arrays only.
- It works by repeatedly dividing the search interval in half.
Iterative Approach
Code
Explanation
- The function
binarySearch
takes an arrayarr
, the size of the arrayn
, and the element to be searchedx
as input. - It initializes two pointers
left
andright
to the start and end of the array respectively. - It iteratively divides the search interval in half by calculating the middle index
mid
. - If the element at the middle index is equal to the element to be searched, it returns the index.
- If the element at the middle index is less than the element to be searched, it updates the left pointer to
mid + 1
. - If the element at the middle index is greater than the element to be searched, it updates the right pointer to
mid - 1
. - If the element is not found in the array, it returns
-1
.
Analysis
- Time Complexity:
O(log n)
wheren
is the number of elements in the array. - Space Complexity:
O(1)
as no extra space is used.
Recursive Approach
Code
Explanation
- The function
binarySearch
takes an arrayarr
, the left indexl
, the right indexr
, and the element to be searchedx
as input. - It calculates the middle index
mid
of the array. - If the element at the middle index is equal to the element to be searched, it returns the index.
- If the element at the middle index is greater than the element to be searched, it recursively searches the left half of the array.
- If the element at the middle index is less than the element to be searched, it recursively searches the right half of the array.
- If the element is not found in the array, it returns
-1
.
Analysis
- Time Complexity:
O(log n)
wheren
is the number of elements in the array. - Space Complexity:
O(log n)
due to the recursive calls.
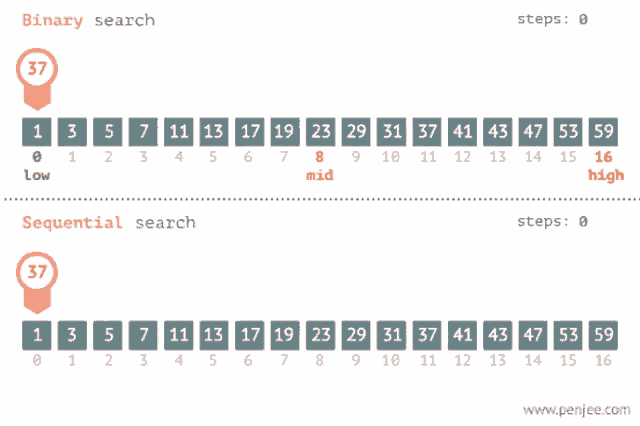